It's easier than ever to get your web app working with a hosted database with MongoDB Atlas. No SQL knowledge is required whatsoever, and the team at MongoDB has built out a clean UI to get your database up and running. So, let's say you follow their instructions, and you have your database. Now, you're probably wondering what you should do next.
Luckily, there exists a fantastic Python distribution called PyMongo that allows developers to read and write from a MongoDB database with just a few lines of code. This would work for web apps that are built with a Python framework (like Django), or even just Python scripts used for testing or building out an MVP. Let's jump right in.
The first thing you'll need is the address for your MongoDB database. To get this, just log in to your MongoDB Atlas account.
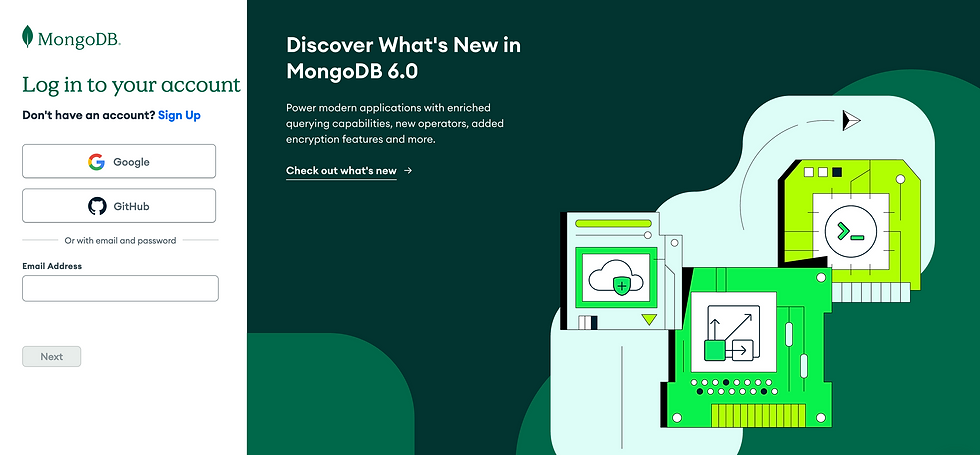
Next, follow the on-screen instructions to set up your first free cluster. As part of this process, MongoDB will prompt you to set a username and password to access the cluster. Make sure to take note of these. The address to access your cluster will look something like this:
mongodb+srv://<your username>:<your password>@<your cluster name>.<your cluster url>.mongodb.net
Great, now that we have that, we can get started with PyMongo in Python. The first line of code you write will be to access your MongoDB cluster using the address. It will look similar to below.
import pymongo
client = pymongo.MongoClient('mongodb+srv://<your username>:<your password>@<your cluster name>.<your cluster url>.mongodb.net')
Perfect. The second thing we do is to grab the database within the cluster that you want to work with. This should've been set up on the MongoDB Atlas site. If you don't remember the name, you can list all databases in Python using the following line.
print(client.list_database_names())
Once we have the database name, we can access it using the following code.
db = client["<your database name>"]
Nice. Now, within the database, we want to choose a collection (similar to the SQL concept of a table). Once again, if you don't remember your collection name you can list them out with a Python command. If you don't have any collections yet, you can just pick any name!
print(db.list_collection_names())
mycollection = db["<your collection name>"]
Adding an entry to a collection is pretty easy. All we need to do is create a dictionary containing all the data we wish to write, and then call a command from PyMongo. See the example code below for reference.
new_entry = {
"name": "My new entry",
"viewed": False,
"id": 7845,
}
res = mycollection.insert_one(new_entry)
print(res.inserted_id)
And that's pretty much it. It's super easy to get started with MongoDB Atlas in Python. For reference, here's all the above code compiled together. Happy coding!
import pymongo
client = pymongo.MongoClient('mongodb+srv://<your username>:<your password>@<your cluster name>.<your cluster url>.mongodb.net')
db = client["<your database name>"]
mycollection = db["<your collection name>"]
new_entry = {
"name": "My new entry",
"viewed": False,
"id": 7845,
}
res = mycollection.insert_one(new_entry)
print(res.inserted_id)