Express (also called Express.js) is a back-end framework written in JavaScript. It's commonly used for writing "RESTful" APIs with Node.js. Just like React is the de facto standard for writing client-side applications, Express is the current developer favorite for the server-side code. React and Express are actually two of the pillars of the MERN stack (MongoDB - Express - React - Node), which is a common set of frameworks used to build web applications. However, remember to never blindly choose frameworks based on a fun acronym and to always select your tools based on the task at hand!
Anyway, to sum that up, Express is the back-end JavaScript sister of React. Express provides React with the data it needs and React shows that data to the user in a nice way. So, the first step to get all of this working is to create an API that React (or any other front-end framework) can call into. Express will help us with this.
Step 1: Create Node Application
The first thing we want to do is create a new directory for our Express app to live, and instantiate a Node app. To do so, open a new terminal window, and execute the following commands.
$ mkdir express-app
$ cd express-app
$ npm init
The npm flow will ask you for your preferences in setting up package.json, and it's okay to accept the defaults for now. Next, we should install Express.
$ npm install express
Step 2: Set Up the API
Let's create a JavaScript file called app.js, and begin designing our API. We can begin by importing the express package, instantiating our app object, and setting the port.
$ touch app.js
-- app.js --
import express from "express";
const app = express();
const PORT = 8080;
The port is what we will use to access our API, either in a browser, from another app, or in a testing tool like Postman. By the way, you should download Postman now. You'll need it shortly. I chose "8080", but you can choose pretty much any number as long as it isn't being used by something else. It's also good to stay from 0 to 1023 because those are considered "system" ports.
Okay, so to make sure our API is ready to accept any requests, we'll add a listener function, and we'll also add a console log so we have some feedback that tells us everything is working properly. That code can go just below the line where you define the port.
-- app.js --
app.listen(PORT, (error) => {
if(!error) {
console.log("The server is running!");
} else {
console.log("An error has occurred.");
}
});
Awesome, now go to the terminal and execute the below node command. If you see "The server is running!" printed out, then everything is working properly!
$ node app.js
Step 3: Implement the API
Cool. Now that the Express API is working, we can add some functions to accept incoming GET requests. Note that GET requests are only one of four different requests: GET, POST, PUT, and DELETE. A simple GET request would return a string of characters. A more complex GET request would accept some information and return something based on the information received. We'll implement both of these. Let's start with the simplest GET request. Let's start all of our API URIs with "/api/v1". We can store this in a variable just below where the port is defined. This will ensure there is no confusion that we're accessing an API and will also allow us to version our API. The full URI for the first route will be "/api/v1/basic". That will be the first parameter passed into the Express get function. Then, we'll set the status to 200 (which means OK). Finally, we'll return our information using the send function.
-- app.js --
const ROOTv1 = "/api/v1";
app.get(`${ ROOTv1 }/basic`, (req, res) => {
res.status(200);
res.send("Here's some information from the server.");
});
After starting your server, let's fire up Postman and make sure everything works properly. Create a new request, set it to GET, and input "localhost:8080/api/v1/basic" as the URI. The, go ahead and click send. At the bottom, in the response, you should see "Here's some information from the server". If you do, that means you just made your first API request to your own API! Congrats!
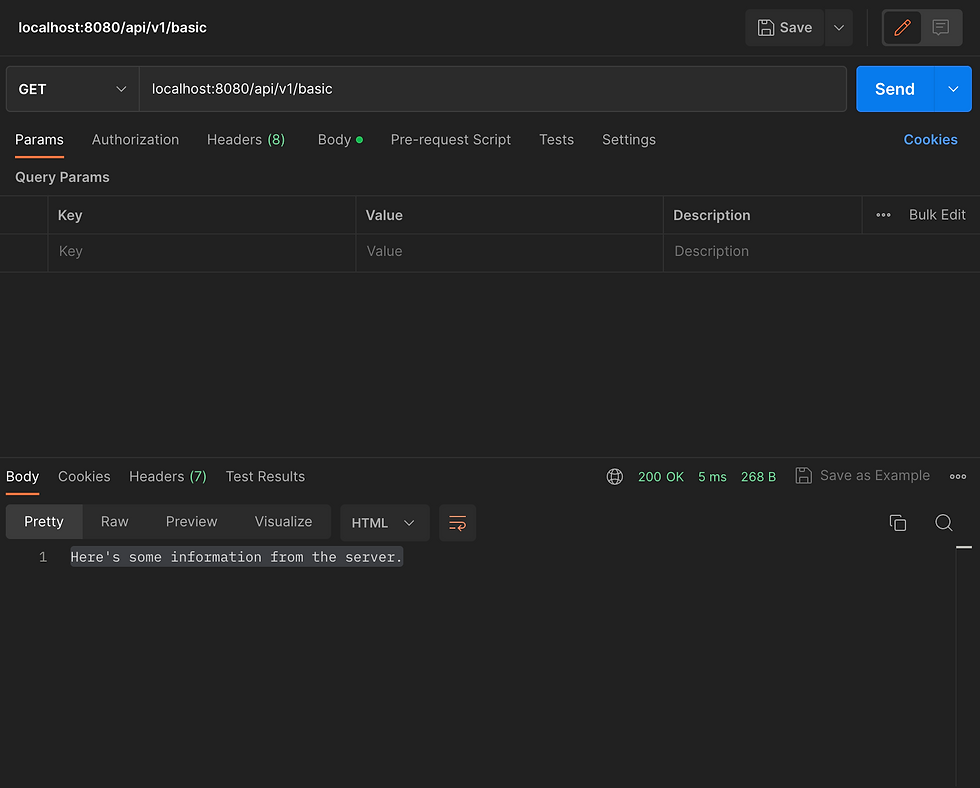
Amazing. Now, let's complicate things a bit. We'll write some code to accept a GET request that includes some useful information in its body (in JSON), parses it, and returns a response based on the received information. It's not as difficult as it sounds. First, we need to configure our Express app to use JSON. We can do that at the top of the file near where the port is set. Then, we use the "req" parameter to access the body, and then the passed in information. Finally, we set the status and send a response just as we did before. For this URI, we'll use the same root, and add "name" to the end.
-- app.js --
app.use(express.json());
app.get(`${ ROOTv1 }/name`, (req, res) => {
const { name } = req.body;
res.status(200);
res.send(`Here's some information from the server based on your name, ${ name }.`);
});
Great. Now, let's start up our server, and open up Postman again. Enter the URI just as you did before (except with "name" instead of "basic", like "localhost:8080/api/v1/name"), and then switch to the "Body" tab. Here, you should enter the JSON data that includes a "name" parameter. See below.
{
"name":"Aaron"
}
Now all you have to do is click send! If you see a response below that includes the name you passed in, everything is working! Good job!
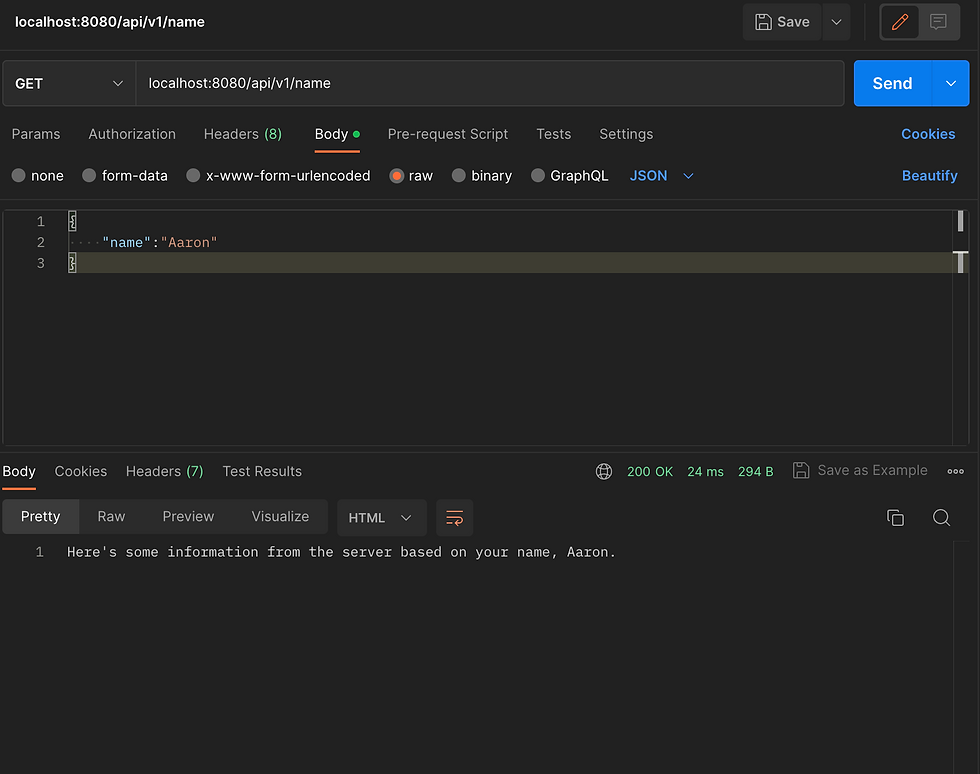
And that's it! You've written a simple API using Express. That wasn't so bad, was it? If you'd like to view all of the code, jump over to our GitHub. Thanks for reading!